Small script: A multi-threaded poll spammer bot
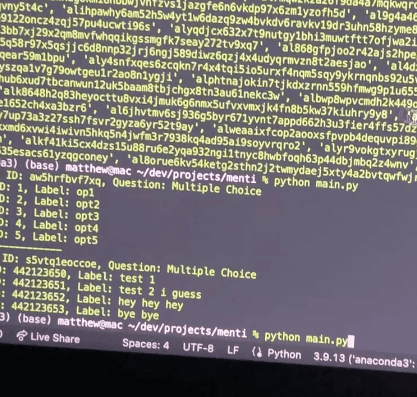
What is this?π
I wrote a Python script to automate menti.com live polls, the highlight being the capability to submit n poles at the same time using multiple threads (for dramatic effect, obviously). It is a very "down-and-dirty" solution, being built quickly for my own personal usage and not meant to be elegant.
Project organizationπ
This bot is structured into two primary Python files: main.py
and api.py
. The former script orchestrates user interaction and directs the flow of the application, while api.py
contains the core functionality that interfaces with and manipulates the Menti API. This separation of concerns allows for a clean codebase where the user interface logic is decoupled from the API interaction logic.
Interacting with the userπ
The main.py
file begins by collecting necessary information from the user via simple input
functions, such as the numeric ID of the Menti poll and the desired number of votes. User input is then passed to api.py
functions to validate and prepare for the voting process.
API integrationπ
The api.py
module employs several HTTP requests to interact with the Menti API. It uses regular expressions (via re
) to scrape the necessary data from the Menti poll's HTML content and the requests
library to handle needed POST and GET requests.
The complete list of API-interacting functions are as follows:
def get_questions(game_code):
...
def get_identifiers(n = 1, also_append_to_file = False):
...
def vote(question_id, vote_id, identifier):
...
def read_uids_from_file(limit = 1, skip = 0):
...
def get_game_id_from_number_code(number_code):
...
def vote_concurrently(uids, question_id, vote_id):
...
Multithreading for dramatic flareπ
To ensure efficiency and speed of voting, vote_concurrently
in api.py
uses Python's ThreadPoolExecutor
to submit multiple votes in parallel. This takes advantage of multithreading to speed up the voting process, a crucial aspect when casting a significant number of votes. If this wasn't used, voting 50 times would take many seconds, however, using threads, it takes < 1 second.
User ID managementπ
The api.py
module generates unique identifiers for each vote to mimic real users. This is achieved through the get_identifiers
function, which can also append identifiers to a specified file for future use, showcasing a use case for those interested in persistent identifier management (AKA: being able to have the same 5 users voting across multiple days and polls).
My first time using the scriptπ
The background music syncing with the video wasn't intentional, but sounds awesome, so I decided to share it!
Disclaimerπ
Like most automation scripts, this is not meant to be used maliciously, but has the capacity for it. This should only be used responsibly. I'm still deciding if I want to make the repository public.